最近在使用ESP32搭建web服务器测试,发现esp32搭建这类开发环境还是比较方便的。具体的http协议这里就不再赘述,我们主要说一下如何使用ESP32提供的API来搭建我们的http web。
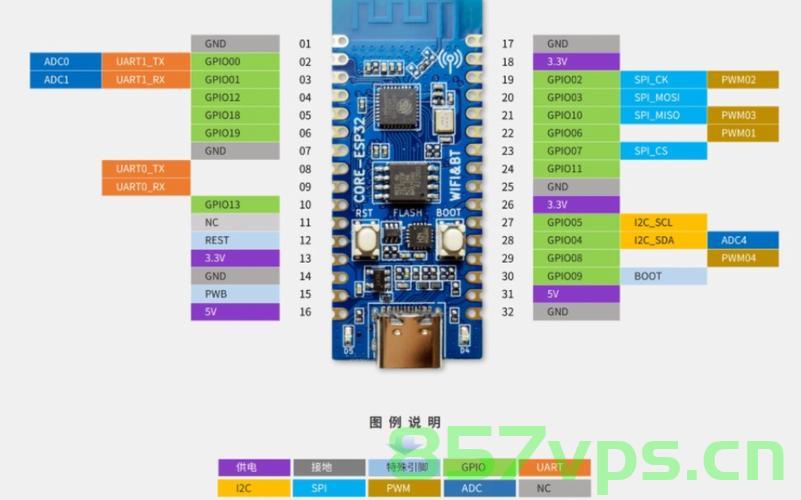
一、web服务器搭建过程
1、配置web服务器
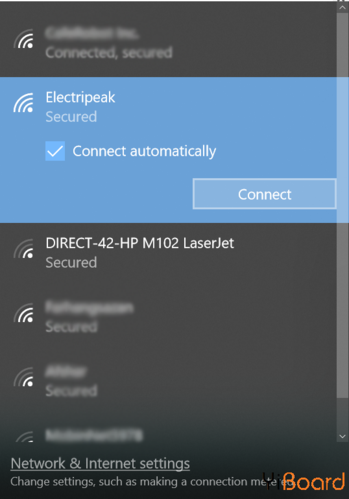
在ESP-IDF中,Web服务器使用httpd组件实现。我们需要先创建httpd_config_t结构体,指定服务器的端口、最大并发连接数、URI匹配处理器等选项。然后,我们通过调用httpd_start函数来启动Web服务器。(使用默认的配置就可以,包括端口号都已经默认配置好了)
httpd_config_t config = HTTPD_DEFAULT_CONFIG(); httpd_handle_t server = NULL; // 设置服务器端口为80 config.server_port = 80; // 创建HTTP服务器句柄 if (httpd_start(&server, &config) != ESP_OK) { printf("Error starting server!\n"); return; }
2、 注册 URI处理器
在Web服务器启动后,我们需要为不同的URI注册处理器函数。当Web服务器接收到请求时,会根据请求的URI选择相应的处理器函数进行处理。在ESP-IDF中,我们可以使用httpd_register_uri_handler函数注册URI处理器。该函数的原型如下
esp_err_t httpd_register_uri_handler(httpd_handle_t hd, const httpd_uri_t *uri)
其中,hd参数为HTTP服务器句柄;uri参数为包含URI路径、HTTP方法、处理函数等信息的结构体指针。例如:
static const httpd_uri_t echo = { .uri = "/", .method = HTTP_POST, .handler = echo_post_handler, .user_ctx = NULL }; static esp_err_t echo_post_handler(httpd_req_t *req) { char buf[100]; // char ssid[10]; // char pswd[10]; int ret, remaining = req->content_len; while (remaining > 0) { /* Read the data for the request */ if ((ret = httpd_req_recv(req, buf, MIN(remaining, sizeof(buf)))) = 0) { alert("File path on server cannot have spaces!"); } else if (filePath[filePath.length-1] == '/') { alert("File name not specified after path!"); } else if (fileInput[0].size > 200*1024) { alert("File size must be less than 200KB!"); } else { document.getElementById("newfile").disabled = true; document.getElementById("filepath").disabled = true; document.getElementById("upload").disabled = true; var file = fileInput[0]; var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (xhttp.readyState == 4) { if (xhttp.status == 200) { document.open(); document.write(xhttp.responseText); document.close(); } else if (xhttp.status == 0) { alert("Server closed the connection abruptly!"); location.reload() } else { alert(xhttp.status + " Error!\n" + xhttp.responseText); location.reload() } } }; xhttp.open("POST", upload_path, true); xhttp.send(file); } } function send_wifi() { var input_ssid = document.getElementById("wifi").value; var input_code = document.getElementById("code").value; var xhttp = new XMLHttpRequest(); xhttp.open("POST", "/wifi_data", true); xhttp.onreadystatechange = function() { if (xhttp.readyState == 4) { if (xhttp.status == 200) { console.log(xhttp.responseText); } else if (xhttp.status == 0) { alert("Server closed the connection abruptly!"); location.reload() } else { alert(xhttp.status + " Error!\n" + xhttp.responseText); location.reload() } } }; var data = { "wifi_name":input_ssid, "wifi_code":input_code } xhttp.send(JSON.stringify(data)); }
static esp_err_t html_default_get_handler(httpd_req_t *req) { // /* Send HTML file header */ // httpd_resp_sendstr_chunk(req, ""); /* Get handle to embedded file upload script */ extern const unsigned char upload_script_start[] asm("_binary_upload_script_html_start"); extern const unsigned char upload_script_end[] asm("_binary_upload_script_html_end"); const size_t upload_script_size = (upload_script_end - upload_script_start); /* Add file upload form and script which on execution sends a POST request to /upload */ httpd_resp_send_chunk(req, (const char *)upload_script_start, upload_script_size); /* Send remaining chunk of HTML file to complete it */ httpd_resp_sendstr_chunk(req, ""); /* Send empty chunk to signal HTTP response completion */ httpd_resp_sendstr_chunk(req, NULL); return ESP_OK; } httpd_uri_t html_default = { .uri = "/", // Match all URIs of type /path/to/file .method = HTTP_GET, .handler = html_default_get_handler, .user_ctx = "html" // Pass server data as context }; httpd_register_uri_handler(server, &html_default);
这里要特别注意:
1)、.uri = "/",这个表示当你打开网页的IP地址后第一个要显示的页面,也就是要放到根目录下("/"也就是根目录)。
2)、ESP32可以直接将html的网页编译进来不需要转为数组,但在CMakeList.txt文件中需要EMBED_FILES upload_script.html这样的形式引入网页文件。这个html编译出出来的文本文件怎么使用呢。wifi.html编译出来一般名称是默认的_binary_名称_类型_start。这个指针代编译出来文件的起始地址。_binary_名称_类型_end,代表结束地址。wifi.html的引用方式如下。通过以下的方式就可以获得html这个大数组。
extern const unsigned char upload_script_start[] asm("_binary_upload_script_html_start"); extern const unsigned char upload_script_end[] asm("_binary_upload_script_html_end"); const size_t upload_script_size = (upload_script_end - upload_script_start); /* Add file upload form and script which on execution sends a POST request to /upload */ httpd_resp_send_chunk(req, (const char *)upload_script_start, upload_script_size);
3、实现URI处理函数
在注册URI处理器后,我们需要实现对应的处理器函数。URI处理器函数的原型为:
typedef esp_err_t (*httpd_uri_func_t)(httpd_req_t *req);
如上面示例中的:
static esp_err_t html_default_get_handler(httpd_req_t *req)
4、处理HTTP请求
在URI处理器函数中,我们可以通过HTTP请求信息结构体指针httpd_req_t获取HTTP请求的各种参数和数据。以下是一些常用的HTTP请求处理函数:
httpd_req_get_hdr_value_str:获取HTTP请求头中指定字段的值(字符串格式)
httpd_req_get_url_query_str:获取HTTP请求URL中的查询参数(字符串格式)
httpd_query_key_value:解析HTTP请求URL中的查询参数,获取指定参数名的值(字符串格式)
httpd_req_recv:从HTTP请求接收数据
httpd_req_send:发送HTTP响应数据
httpd_resp_set_type:设置HTTP响应内容的MIME类型
httpd_resp_send_chunk:分块发送HTTP响应数据。
例如,以下是一个URI处理器函数的示例,用于处理/echo路径的POST请求:
static esp_err_t echo_post_handler(httpd_req_t *req) { char buf[1024]; int ret, remaining = req->content_len; // 从HTTP请求中接收数据 while (remaining > 0) { ret = httpd_req_recv(req, buf, MIN(remaining, sizeof(buf))); if (ret content_len); httpd_resp_send_chunk(req, NULL, 0); return ESP_OK; }
5、 发送响应
在URI处理函数中,可以使用httpd_resp_send()函数将响应发送回客户端。该函数需要传入一个httpd_req_t结构体作为参数,该结构体表示HTTP请求和响应。
例如,在上面的hello_get_handler处理函数中,可以使用httpd_resp_send()函数将“Hello, World!”字符串作为响应发送回客户端:
static esp_err_t hello_get_handler(httpd_req_t *req) { const char* resp_str = "Hello, World!"; httpd_resp_send(req, resp_str, strlen(resp_str)); return ESP_OK; }
二、主要使用API的说明
1. httpd_register_uri_handler
用于将HTTP请求的URI路由到处理程序。这个函数接收两个参数:httpd_handle_t类型的HTTP服务器句柄和httpd_uri_t类型的URI配置。
2. httpd_handle_t
httpd_handle_t是HTTP服务器的一个句柄,它是通过httpd_start函数创建的。而httpd_uri_t则定义了HTTP请求的URI信息,包括URI路径、HTTP请求方法和处理函数等。
3. httpd_query_key_value获取变量值
httpd_query_key_value 用于从查询字符串中获取指定键的值。查询字符串是指URL中?后面的部分,包含多个键值对,每个键值对之间使用&分隔。例如,对于以下URL:
http://192.168.1.1/path/to/handler?key1=value1&key2=value2
获取其中的:
esp_err_t httpd_query_key_value(const char *query, const char *key, char *buf, size_t buf_len);
这是一个使用示例
char query_str[] = "key1=value1&key2=value2"; char key[] = "key1"; char value[16]; if (httpd_query_key_value(query_str, key, value, sizeof(value)) == ESP_OK) { printf("value=%s\n", value); } else { printf("key not found\n"); }
4. 获取get参数示例
下面定义的 handler 演示了如何从请求参数里解析 字符串param1和整型变量param2:
esp_err_t index_handler(httpd_req_t *req) { char* query_str = NULL; char param1_value[10] = {0}; int param2_value=0; query_str = strstr(req->uri, "?"); if(query_str!=NULL){ query_str ++; httpd_query_key_value(query_str, "param1", param1_value, sizeof(param1_value)); char param2_str[10] = {0}; httpd_query_key_value(query_str, "param2", param2_str, sizeof(param2_str)); param2_value = atoi(param2_str); } char resp_str[50] = {0}; snprintf(resp_str, sizeof(resp_str), "param1=%s, param2=%d", param1_value, param2_value); httpd_resp_send(req, resp_str, strlen(resp_str)); return ESP_OK; }
5. 获取post参数示例
下面的示例代码中根据httpd_req_t的content_len来分配一个缓冲区,并解析请求中的POST参数:
esp_err_t post_demo_handler(httpd_req_t *req) { char post_string[64]; int post_int=0; if (req->content_len > 0) { // 从请求体中读取POST参数 char *buf = malloc(req->content_len + 1); int ret = httpd_req_recv(req, buf, req->content_len); if (ret content_len] = ''; // 解析POST参数 char *param_str; param_str = strtok(buf, "&"); while (param_str != NULL) { char *value_str = strchr(param_str, '='); if (value_str != NULL) { *value_str++ = ''; if (strcmp(param_str, "post_string") == 0) { strncpy(post_string, value_str, sizeof(post_string)); } else if (strcmp(param_str, "post_int") == 0) { post_int = atoi(value_str); } } param_str = strtok(NULL, "&"); } free(buf); } // 将结果打印输出 printf("post_string=%s, post_int=%d\n", post_string, post_int); // 返回成功 httpd_resp_send(req, NULL, 0); return ESP_OK; } httpd_uri_t post_uri = { .uri = "/post", .method = HTTP_POST, .handler = post_demo_handler, .user_ctx = NULL };
还没有评论,来说两句吧...